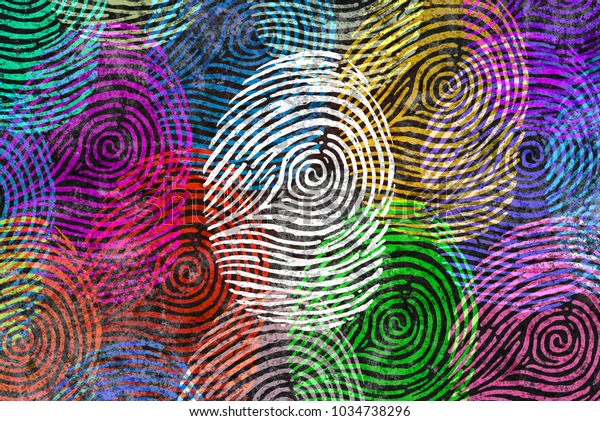
Remember when we talked about why not use lists as default function arguments because once you change them in the function, the change will always stay with the function? The reason for that was that lists are mutable objects. You can change the information content of lists without changing their identity. This property is called mutability. To understand mutability better, we need to dig up a little bit and look into the nuts and bolts of objects in Python. Understanding the role of the id of objects in Python is the key to understanding mutability.
1- The id() function
When you introduce an object in Python, you give it a name, and whenever you want to refer to that object later, you use that name. Python also needs to distinguish your object from others. So it assigns a name for that object. Since computers like numbers so much, Python uses an integer as the name. This integer is called the id of the object. The id remains the same for as long as the object exists. We can get the id by id() function.
a = 1
print(id(a)) #prints the id of a which is an iteger like: 140526364059952
2- id and mutability
Now that we know how to get the id of an object, we can investigate the mutability. Let me first ask you two questions:
1- Will appending an element to a list change the list’s id?
2- Will changing the value of a variable that contains a number change its id?
Let’s answer these questions. In the following code block, we introduce a list, get its id, append a value to it, and compare the ids before and after appending the value. If the ids are the same, we print ‘same id’ if they are different, we print ‘different ids’
a = [5]
id_before_change= id(a)
a.append(3)
id_after_change = id(a)
print('same id') if id_before_change == id_after_change else print('different ids') # the result is same id
By running this code block, we found out that the id of a list doesn’t change by appending a value to it. Now we repeat it for an integer.
b = 5
id_before_change= id(b)
b = 3
id_after_change = id(b)
print('same') if id_before_change == id_after_change else print('different ids') # the result is different ids
This time the id has changed. Changing the id means that the variable has died and a new variable has substituted it. So, when you append an item to a list, you keep the list alive, while when you change the value of a numeric variable, you delete that variable and introduce a new variable with the same name.
The objects whose ids can remain constant while changing their information content are called mutable, and objects that change the id by changing their information content are called immutable.
Lists, dictionaries, and sets are mutable objects.
ints, strings, floats, and tuples are immutable objects.
3- Mutability and hashing
Hashing represents a large amount of data into a fixed-size string, a byte array, or an integer. It is deterministic, i.e. for the same data you always have the same hash.
Python uses hashing extensively in the dictionary data type. When making a dictionary, each key is hashed. Then the hashes and the values are put in a table. This is why sometimes dictionaries are called hash tables.
The Python hash() function does not accept mutable objects. Thus, if you want to check the mutability of an object you can use the hash() function on it.
a = 'this is a string to show how hashing represents data in integer form'
hash(a) #-6941238720518932997
If we try to hash a mutable variable, we get an error.
# Mutable types are not hashable
b = [1]
hash(b)
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
/var/folders/q7/t0v848hn38z8qy436thrkzhc0000gp/T/ipykernel_80922/3237272192.py in <module>
1 # The mutable types are not hashable
2 b = [1]
----> 3 hash(b)
TypeError: unhashable type: 'list'
In Python, you can use mutable objects such as integers, floats, tuples, and strings as the dictionary’s keys, but you cannot use a list or a set as the dictionary keys.
I have another question. What do you think about functions? Are functions mutable? You can answer this question by the methods we learned in this article.
You can access the source codes of this article and other articles here. If you enjoyed reading this article as much as I enjoyed writing it, please give me a star on GitHub.